I attended Hoover Middle School during the mid 80s and remember my first experience instructing a computer to do something. My school was located in a fairly affluent area of Albuquerque, New Mexico. Albuquerque is the home of Sandia National Laboratories and the public education system there seemed to benefit by having quality technology and science programs. In 7th grade I had a brief chance to learn an introduction to programming on an Apple II with a color monitor. This was an amazing opportunity for the time considering desktop computing was just becoming available to the public! The API was a heavily wrapped version of BASIC that allowed students to write lines of code to control the color of pixels on what was probably a 50×50 grid with a 16 color palette. There were very few commands at the disposal of the students and I imagine the code would have looked something like this:
10 clear white
20 set 4,6 blue
30 set 6,6 blue
40 set 3,4 green
50 set 4,3 green
60 set 5,3 green
70 set 6,3 green
80 set 6,4 green
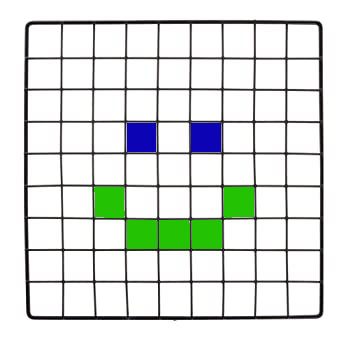
I remember it was fun to write instructions and create something visual on the screen, but the simplicity of the learning experience did not leave me feeling inspired. As an Eldorado High School freshman, my next programming experience was undertaken to impress Rhonda, a beautiful junior cheerleader. We shared a biology class and I had acquired some useful information about an upcoming multiple choice test. My family owned an MSDOS (pre windows) Leading Edge PC with a proprietary word processor and floppy disk drive. I wanted to print a very small cheat sheet for the test that one could literally digest if compelled to do so, but the options available were too limited. Around this time my mother had just purchased a new printer and asked if I could figure out how to configure it. I remembered a page in the manual showcasing the font sizes available and some associated BASIC code to manipulate the printer from DOS. This would be perfect for my test guide, so I learned a great deal about making my way around DOS, directory structures, file editing and programming in BASIC. My first independently conceived program worked; I shared my tiny test guide with Rhonda and was rewarded with a hug, smile, and a little respect. I had seen some potential for the value of programming and began to tinker more with writing BASIC programs.
Another interest I had at the time was in amateur electronics. I grew up designing and building model train sets which gave me an early introduction into how electricity works. All of these technical hobbies provided a fruitful connection to my father who has EE undergraduate degrees and a PHD in computer science. One weekend, he was teaching me about resistors and how to decode their color bands to identify their value. Each color relates to a whole number from 0-9, with gold and silver indicating the quality or tolerance of the resistor. The first two color bands represent the base value and the third indicates the power of 10 to multiply by.
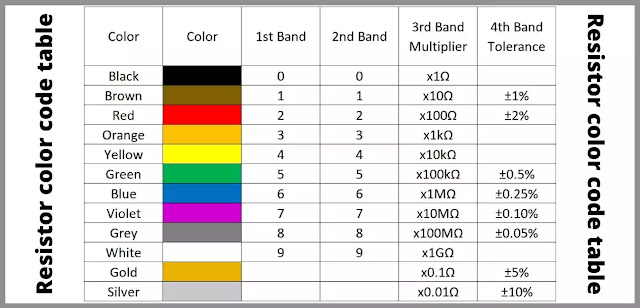
For example, the top resistor pictured on the right has a gold band indicating a 5% tolerance, with three color bands Green(5), Black(0) and Red(2).
This relates to 50*10^2 = 5000 Ohms.
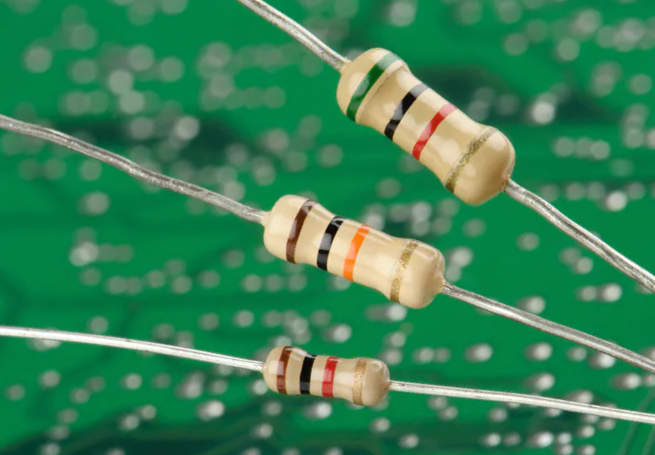
Selecting a resistor for your project can be a bit trickier because you will not always find a perfect match for the exact value you would need. At times selecting the resistor with the closest value would have to do. After the weekend with my dad, I returned home and wrote a program called “Resistance Assistance” to aid in choosing a resistor. The input of the program was a theoretical resistance value and the output was the closest physical resistor and it’s color code.
I had my program on a 720 KB floppy disc the next time I visited my dad and showed him how it worked. He was proud of the way the program performed and was immediately interested in the code I had written. We spent the following couple of hours examining and refining the code to a more professional standard. It was an experience that impacted my personality as a programmer immensely. I will illustrate the progression of refinement to my code with four examples.
#1 Minimizing Logical Statements
The following lines assign the variable C$ variable to the color that corresponds to the correct color. The code is logically accurate but poorly written. At the time I was unaware of the ELSE statement. Even though the correct color will be assigned in line 40, every following comparison statement after will be run resulting in 7 unnecessary calls.
10 NUMBER=2
20 IF NUMBER=0 THEN C$="BLACK"
30 IF NUMBER=1 THEN C$="BROWN"
40 IF NUMBER=2 THEN C$="RED"
50 IF NUMBER=3 THEN C$="ORANGE"
60 IF NUMBER=4 THEN C$="YELLOW"
70 IF NUMBER=5 THEN C$="GREEN"
80 IF NUMBER=6 THEN C$="BROWN"
90 IF NUMBER=7 THEN C$="VIOLET"
100 IF NUMBER=8 THEN C$="GRAY"
110 IF NUMBER=9 THEN C$="WHITE"
120 PRINT "COLOR " C$
My dad described this problem to me and helped me to imagine this part of the program running hundreds, thousands, or even millions of times. If the number variable was evenly distributed between 0 and 9, the average number of calls wasted would be 5 per run. This would cause noticeable slowdown. We refined the code to include ELSE statements so that once a color was assigned the following IF statements would be ignored. Instead of 10 comparisons being made in the code above, the following only requires 3.
10 NUMBER=2
20 IF NUMBER=0 THEN C$="BLACK" ELSE
30 IF NUMBER=1 THEN C$="BROWN" ELSE
40 IF NUMBER=2 THEN C$="RED" ELSE
50 IF NUMBER=3 THEN C$="ORANGE" ELSE
60 IF NUMBER=4 THEN C$="YELLOW" ELSE
70 IF NUMBER=5 THEN C$="GREEN" ELSE
80 IF NUMBER=6 THEN C$="BROWN" ELSE
90 IF NUMBER=7 THEN C$="VIOLET" ELSE
100 IF NUMBER=8 THEN C$="GRAY" ELSE
110 NUMBER=9 THEN C$="WHITE"
120 PRINT "COLOR " C$
#2 Using Data Structures
Next, he showed me how the logic structure could be removed entirely and the same task of assigning a color could be accomplished in one line. He introduced me to the method of using an indexed array for the task. First, store the colors in a list (A$) and then simply access the needed color by location (line 50). There would be some overhead to loading the data in lines 10-30, but this would only need be done once in the program. From that point forward, a million color assignments could be made with a minimal million calls as in line 50.
10 DIM A$(10)
20 FOR I=1 TO 10: READ A$(I):NEXT
30 DATA "BLACK","BROWN","RED","ORANGE","YELLOW",
"GREEN","BROWN","VIOLET","GRAY","WHITE"
40 NUMBER=2
50 C$ = A$(NUMBER)
#3 User Friendliness
The original code I had written worked so long as the resistance values entered were strictly positive integers between one and a billion. What if a user entered a negative value or a non-numeric like the word “dog”? The program would crash. My dad explained that even though we programs new not to do this, we should catch this input error and give the user information and another chance. Also, there should be a way to gracefully exit the program. So, we added a few more lines. Line 120 let the user know how to exit the program and we catch that request on line 140 by exiting back to DOS if entered. Line 150 ensures that the resistance values entered for conversion are in a specified range. If not, the user will be reminded of the limitation and get another shot. These changes made the program more user friendly.
120 PRINT " PRESS F1+ENTER TO QUIT RESISTENCE ASSISTENCE"
130 INPUT;" ▐▐▐▐▐▐ ENTER DESIRED RESISTENCE 9 ",N
140 IF N=-1 THEN SYSTEM
150 IF N=0 OR N<.1 OR N>=1E+11 THEN PRINT" OOPS! BETWEEN .1 AND 9.54E+10 PLEASE.":GOTO 130
#4 Math can often reduce code
The final step we took with the “Resistance Assistance” program was to make it an actaul tool by employing some mathematics. My assumption upon writing the original code was that a resistor could be found for any value needed. I didn’t stop to think that manufacturers probably couldn’t effectively make billions of different sizes. Prior to my birth, the IEC (International Electrotechnical Commission) created a limited set of resistor values to span the predicted need. (…more). The available resistor values are based on a logarithmic scale, which at the time, I didn’t really understand. So, my dad gave me some quality explanation to help me understand and then integrated the mathematics to speed up and reduce the size of the program once again. This was neat. I had always liked math and really had my eyes opened to how interesting programming could be.
It may have been that day, or during some later conversations, when I heard my dad describe his appreciation for “Elegant Code.” With the interest and patience of a great teacher, he helped refine my rough attempt at solving a problem into something more beautiful. I have come to truly enjoy the process of algorithm refinement. Like a sculptor working to reveal a form that awaits under the stone, I chip away at my rough code until the elegant solution remains. I have been hooked since. Thanks Dad!
Below is the final GWBasic Code
10 KEY OFF
20 CLS
30 KEY 1, "-1"
40 OPTION BASE 1
50 DIM B(25),R(24),L$(12)
60 FOR I=1 TO 24:READ B(I):NEXT
70 DATA 1.05,1.15,1.25,1.4,1.55,1.7,1.9,2.1,2.3,2.55,2.85,3.15,3.45,3.75,4.05,4.45,4.9,5.35,5.9,6.5,7.15,7.85,8.65,9.55
80 FOR I=1 TO 24:READ R(I):NEXT
90 DATA 1,1.1,1.2,1.3,1.5,1.6,1.8,2,2.2,2.4,2.7,3,3.3,3.6,3.9,4.2,4.7,5.1,5.6,6.2,6.8,7.5,8.2,9.1
100 FOR I=1 TO 12:READ L$(I):NEXT
110 DATA "SILVER","GOLD","BLACK","BROWN","RED","ORANGE","YELLOW","GREEN","BLUE","VIOLET","GRAY","WHITE"
120 PRINT " PRESS F1+ENTER TO QUIT RESISTANCE ASSISTANCE"
130 INPUT;" ▐▐▐▐▐▐ ENTER DESIRED RESISTANCE 9 ",N
140 IF N=-1 THEN SYSTEM
150 IF N=0 OR N<.1 OR N>=1E+11 THEN PRINT" OOPS! BETWEEN .1 AND 9.54E+10 PLEASE.":GOTO 130
160 P=INT(LOG(N)/LOG(10))
170 X=N/(10^P)
180 FOR I=1 TO 24
190 IF X<B(I) THEN A=R(I):GOTO 220
200 NEXT
210 X=X/10:P=P+1:GOTO 180
220 IF P<3 THEN J$="":T=P:GOTO 250
230 IF P>=3 AND P<6 THEN T=P-3:J$="k":GOTO 250
240 IF P>=6 THEN T=P-6:J$="M"
250 N=A*(10^T)
260 BEEP
270 H$=L$(INT(A)+3)+" ║ "+L$(10*(A-INT(A))+3)+" ║ "+L$(P+2)
280 Z$="▐───────────────────"
290 Q=LEN(H$)
300 K$=LEFT$("▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄",Q+4)
310 D$=LEFT$("▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀",Q+4)
320 PRINT" ☺ USE A";N J$" Ω RESISTOR
330 PRINT" "K$
340 PRINT" ───────────────────▌ "H$" "Z$"
350 PRINT" "D$:GOTO 130